This example code is a simple example showing how the MFC classes can be used to wrapper the HTTP API calls and retrieve product information. This example is retrieving a single product and displaying rudimentary details. Calling the API in this way has no dependencies on libraries.
If you do not program using C++/MFC this example still shows the overall logic that would be applicable to most languages
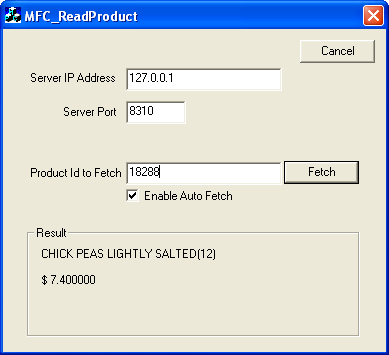
This code is deliberately simple and would need enhancing before use in production level code
- The code uses the returned XML string without using an XML parser
- The network call is made on the main thread and will block screen updates while waiting for a response on the network
- Network errors are displayed in technical form to the user
Code
Download Visual Studio Project (37Kb)// Read user interface, [not shown here, download full source] long thePid = ???; // Value from User Interface // Build the URL we want CString Url; // For this example we request Named XML format Url.Format("/gnap/M/buck?3=retailmax.elink.products&9=f100=%ld", thePid); // Other formats are possible... // Numeric XML // Url.Format("/gnap/buck?3=retailmax.elink.products&9=f100=%ld", thePid); // Named JSON // Url.Format("/gnap/J/buck?3=retailmax.elink.products&9=f100=%ld", thePid); // Numeric JSON // Url.Format("/gnap/j/buck?3=retailmax.elink.products&9=f100=%ld", thePid); CString gError; CString ReplyString; try { CInternetSession sess("MFC_ReadProduct " __DATE__ " " __TIME__); CHttpConnection* pHttp = sess.GetHttpConnection(m_ServerAddress, (INTERNET_PORT) port); if (pHttp) { const char* Accept[2] = { "*/*", NULL }; CHttpFile* pHFile = pHttp->OpenRequest("GET", Url, NULL, 1, (const char**) Accept); if (pHFile) { pHFile->SendRequest(); DWORD Status = 0; pHFile->QueryInfoStatusCode(Status); if ((Status >= 200) && (Status <= 299)) { { char bb[4096]; int readlen = pHFile->Read(bb, sizeof(bb)); while (readlen > 0) { ReplyString += CString(bb, readlen); readlen = pHFile->Read(bb, sizeof(bb)); } } } else { switch (Status) { case 404: gError += "URL or file not found"; break; default: CString w; w.Format("Request to server failed with error=%ld. Read failed", Status); gError += w; } } delete pHFile; } delete pHttp; } } catch (CInternetException*pI) { char bb[4096]; pI->GetErrorMessage(bb, sizeof(bb)); gError += "Network level error:\n\n"; gError += bb; pI->Delete(); } if (! gError.IsEmpty()) { AfxMessageBox(gError, MB_ICONSTOP); return; } // Display raw response string AfxMessageBox(ReplyString); // Decode and display response. [not shown here, download full source]