Generic eCommerce Interface
If you are a website dealing with shoppers, the Generic eCommerce interface allows you to provide URL endpoints that a Fieldpine system will call out to in order to communicate.
- Fieldpine Systems call out to your website (polling method).
- Fieldpine push products to you at regular intervals
- Sales are polled at regular intervals from a URL you provide
- Website is responsible for securing the interface endpoints.
- Suitable for low to medium volumes
Getting Started
To implement a new generic eCommerce interface, follow these suggested steps.
- Get the bones of the website running
- Create an endpoint to receive product details. Ask the client retailer to start sending information to this end point (How to Configure)
- Implement processing of the inbound products
- Create an endpoint to retrieve sales information.
- Test this endpoint yourself using a browser interactively
- When you are happy it is functional, consider asking Fieldpine Support to validate your output
- When ready, ask the retailer to start polling this endpoint for sales.
- This is now an operational eCommerce interface. Extend out with any additional requirements you require.
Products Upload
Create a URL endpoint on your website. At regular intervals Fieldpine will post all the relevant products to this URL.
POST /upload/Products HTTP/1.1 Content-Length: 33452 Content-Type: application/xml X-Fieldpine-RmSystem: 5,78,22,11 <ARAY> <DATS> <Pid>123</Pid> <Description>Can of food</Description> <Unitprice>3.99</Unitprice> ...
Products are pushed in XML format
Products Example
<ARAY> <DATS> <Pid>123</Pid> <Description>Can of food</Description> <Unitprice>3.99</Unitprice> ... </DATS> <DATS> <Pid>456</Pid> <Description>15W 40 Oil. 4L</Description> <Unitprice>31.50</Unitprice> ... </DATS> </ARAY>
Test your Products URL now
Sales Download
Create a URL endpoint on your website that responds with sales records. At regular intervals Fieldpine will poll this endpoint. You do not need to worry about repeating a sale that has already been sent, the Fieldpine side will remove any duplicates it already has.
Details about URL structure
If your endpoint does not understand query parameters (ie if for /getsales.xml?a=b it ignores the ?a=b portion), then you should respond with all sales completed in the last 72 to 120 hours (3 to 5 days). This ensures that delays in polling does not cause undue sales loss. You may send older sales if you prefer. Do not assume that a sale that has been "polled" has been processed, we may poll for a sale and fail to process it for some reason.
If your endpoint does understand query parameters then the following query parameters may be seen:
The expected equivalent in SQL is select * from sales where end-date-time >= date-time order by date-time
The expected equivalent in SQL is select * from sales where end-date-time < date-time order by date-time
Your endpoint should be able to handle the following sequence if it is functioning correctly. Assume your first ever sale was made on 13-Jan-2014 9:42:33, and sales were then completed one per calendar day after that
GET /getsales.xml?before=1-jan-2010 00:00:00&limit=1 -- Respond with empty set GET /getsales.xml?before=1-jan-2015 00:00:00&after=1-jan-2010 00:00:00&limit=1 -- Respond with single sale from 13-Jan-2014 9:42:33 GET /getsales.xml?before=1-jan-2015 00:00:00&after=13-jan-2014 9:42:00&limit=100 -- Respond again with sale from 13-Jan-2014 9:42:33 and a further 99 sales.
Notice how in the third query the server backtracks time slightly. This is to ensure that if multiple sales complete at exactly the same instant they will all be fetched. If you honour the date selectors correctly you do not need to worry about duplicates.
Response Packet Format
TDB.A response packet should include an outer level <ARAY> packet with individual sales inside.
The response for "No Sales" is simply an empty XML response
<ARAY></ARAY>
If you encounter an error and cannot return a reliable result, abort the connection and return nothing. Do not return empty XML as an error indicator
Inside the ARAY, can be one or more SALE packets. These are easiest to understand with examples
<ARAY> <SALE> <ExternalId>ABC1354</ExternalId> <CompletedDt>17-May-2012 14:47:32</CompletedDt> <Phase>200</Phase> <ITEM> <Pid>7824</Pid> <Qty>2</Qty> <TotalPrice>15.00</TotalPrice> <ITEM> <PAYM> <Type>2</Type> <Amount>15.00</Amount> <Ref>18292464</Ref> <PAYM> </SALE> </ARAY>
Example
GET /download/Sales HTTP/1.1 Content-Length: 0 Content-Type: application/xml X-Fieldpine-RmSystem: 5,78,22,11 Your server responds with HTTP/1.1 200 OK Content-Length: 9901 Content-Type: application/xml X-Fieldpine-RmSystem: 5,78,22,11 <-- Optional <ARAY> <SALE> <ExternalId>ABC123</ExternalId> <CompletedDt>17-May-2012 14:47:32</CompletedDt> <Phase>200</Phase> <ITEM> <Pid>7824</Pid> <Qty>2</Qty> <TotalPrice>15.00</TotalPrice> <ITEM> ...
Notes
- Each sale you return MUST include a unique sale identifier in the "ExternalId" field. This identifer must be unique over time and server. If the same id is ever seen twice, the server will consider them to be exact duplicates. Identifers may be up to 32 ascii characters
- Items and payments must balance exactly. Sales that do not balance may not be loaded.
- Do not return full credit card numbers in any field. Doing so would may invoke PCI/DSS requirements. You may send the obscured form safely. If full credit card numbers are detected, the server may choose to stop communicating with you
Security Considerations
Information contained in sales records is likely to include personal customer information, such as name, email, address. You should pay some attention to securing this information.
- You may use HTTP and/or HTTPS to secure in-flight data
- You should consider locking the end-points to a single fixed IP address. Retailers will often
always call from a single IP address
Recommendation: Lock the endpoints to specific IP addresses if the retailer has a fixed IP
- Requests from Fieldpine will always include the HTTP Header X-Fieldpine-RmSystem. This value
is a constant for a given retailer environment. Different retailers and even "test" and "live" environments
in the same retailer will have different values. If this header is NOT present, then the request does not
come from a Fieldpine System. This header does not prove that you are communicating with the correct server,
anybody can place this header in a request.
Recommendation. Always check that X-Fieldpine-RmSystem is the same constant value
- If the retailer has an incoming email address allocated to the Retail System (ie the computer has its own email address), then you can send sales information by email instead if you prefer
How to Configure on Retailers System
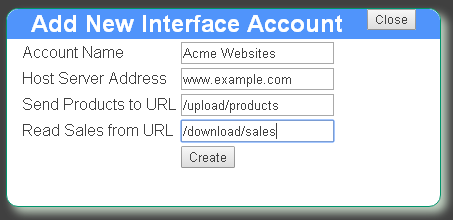
The retailer can enable your external website by selecting "eCommerce" from the main report page and then "General Purpose Interfaces". On the control screen, there is an option to add new interfaces, which can be completed as per the sample shown. The actual configuration screen may have more options than shown in this example, but the example shows the main parts of interest.
The URL given in the "Send Products to URL" will receive the products in the format described above under the section Products Upload
The URL given in the "Read Sales from URL" will be read periodically to check for new sales. This URL should respond as documented above in the section Sales Download
Using AutoConfigure
GET /your-url.htm HTTP/1.1 Content-Length: 0 Host: example.com X-Fieldpine-RmSystem: 5,78,22,11
The AutoConfigure API allows you to supply a single end point that defines how Fieldpine is to interact with your web service.
Fieldpine connects to your endpoint and requests the details of the endpoints and services you require. This means you can dynamically change your interface operation without needing to manually reconfigure the retailers system.
To use autoconfigure, you enter the configuration URL into the retailers system once and then everything else is dynamic
At various intervals, Fieldpine will connect to your configuration URL using a GET request. Your response should consist of a response packet in either XML or JSON.
Response
<ARAY> <EndPoint> <URL>http://server2.example.com/upload/products.htm</URL> <Schedule>4 hours</Schedule> <Purpose>Products</Purpose> </EndPoint> <EndPoint> <URL>http://server2.example.com/upload/sales.htm</URL> <Schedule>1 hour</Schedule> <Purpose>Sales</Purpose> </EndPoint> <EndPoint> <URL>http://server2.example.com/upload/inventory.htm</URL> <URL>http://server3.example.com/upload/inventory.htm</URL> <Schedule>1 hour</Schedule> <Purpose>StockLevels</Purpose> </EndPoint> <ARAY>